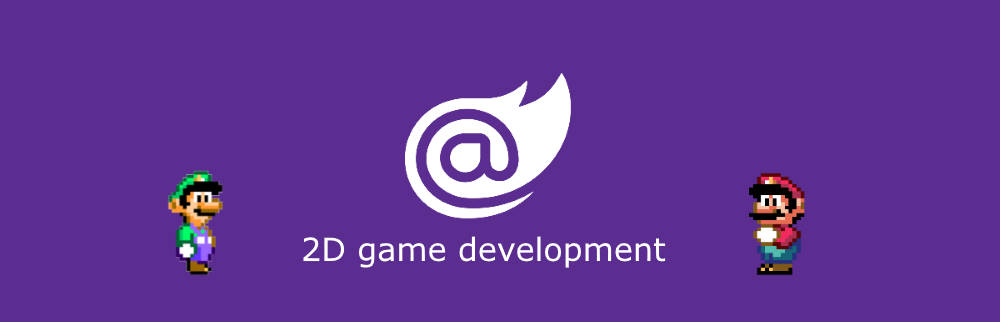
Blazor GameDev – part 3: sprite rendering
Hi All! Welcome to part 3 of the Blazor 2d Gamedev series. Today we’re going to see how to render a sprite and handle the window resize event.
Last time we saw how easy it is to initialize the HTML Canvas and render some text. Now we’re going to expand that code and add the new functionalities.
All the sources are available in the Example 2 folder on GitHub. There’s a demo online as well, available here.
The first thing to do is to update our index.html and add the code to handle window resizing:
function gameLoop(timeStamp) { window.requestAnimationFrame(gameLoop); game.instance.invokeMethodAsync('GameLoop', timeStamp, game.canvas.width, game.canvas.height); } function onResize() { if (!window.game.canvas) return; game.canvas.width = window.innerWidth; game.canvas.height = window.innerHeight; } window.initGame = (instance) => { var canvasContainer = document.getElementById('canvasContainer'), canvases = canvasContainer.getElementsByTagName('canvas') || []; window.game = { instance: instance, canvas: canvases.length ? canvases[0] : null }; window.addEventListener("resize", onResize); onResize(); window.requestAnimationFrame(gameLoop); };
Few things going on here:
- we’re attaching a handler to the window resize event
- in onResize() we store the new window size
- we’ve updated the call to GameLoop() to receive the new size
The next step is to update the Razor page. First we have to add the image to render:#
<img @ref="_spritesheet" hidden src="assets/blazor.png" />
The hidden attribute is necessary to…well…hide the image, otherwise will be displayed right before the canvas.
Lastly, we have to update our GameLoop() function and render the sprite:
@code { ElementReference _spritesheet; [JSInvokable] public async ValueTask GameLoop(float timeStamp, int width, int height) { await _context.ClearRectAsync(0, 0, width, height); await _context.DrawImageAsync(_spritesheet, 0, 0, width/2, height/2); } }
Since we now know the exact window size (and our canvas is set to fill 100%), we can pass the right parameters to ClearRectAsync() . Then we can call DrawImageAsync() passing the sprite reference, position and size.
Et voila!
The next time we’ll see how to move this sprite on the screen. Bye!