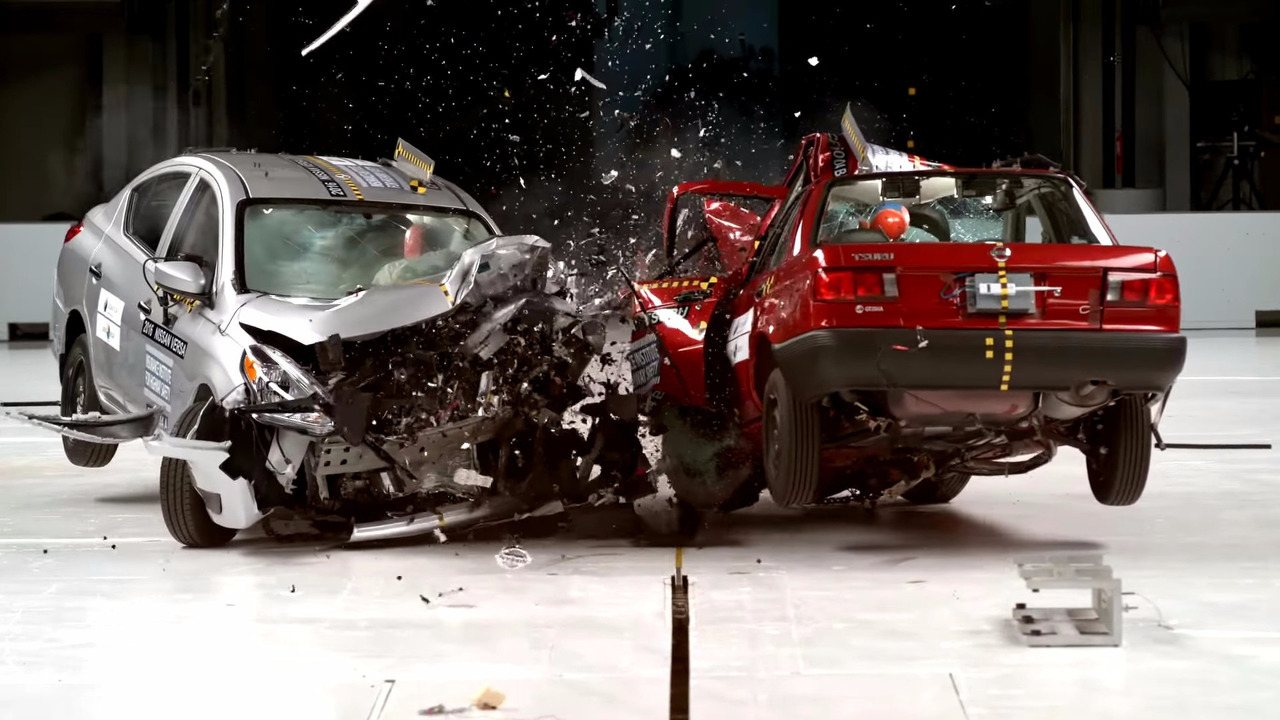
Write integration tests on MongoDB with .NET Core and Docker
Hi All! In this post, we’re going to explore a simple but effective way to write integration tests on MongoDB using .NET Core and Docker.
A while ago I wrote a small series about Unit Testing on MongoDB. A lot has changed since then and I also received few requests to add more details.
To be fair, I am not even that happy now with those articles. I bet it’s the same feeling we’re all experiencing when we look at the code we wrote in the past.
</figure>
Let’s just say that when it comes to testing, we should all follow the Test Pyramid.
And when it comes to the persistence layer, I think the best way is to always “hit the metal” and check if a real DB is happy with the code we wrote.
For example, EntityFramework exposes an In-Memory provider that can be used to write tests. It’s a valuable tool, but it doesn’t guarantee that the DbContext configuration and the Entities mapping is 100% valid.
For that the only thing you can do is bite the bullet, connect to a DB instance, and run few statements.
Now, I wrote already how to write integration tests in a CI/CD pipeline. We are going to use the exact same approach, but for MongoDB instead.
All the code is as usual available on GitHub, feel free to take a look before moving on.
As you can see, I have added a docker-compose configuration to the root. The first step before launching the test suite is to run docker-compose up
. It will download the official MongoDB image if you don’t have it already, and spin up the DB server.
The instance will be available on localhost, port 27097, and this is where we’re pointing our code.
Adding a Docker configuration is something that I’ve been recently doing for basically all my projects: Devs should be able to spin up all the required infrastructure with 1-2 console commands, without spending too much time wondering why things don’t work.
This doesn’t mean we shouldn’t be aware of what is going on under the hood. All the opposite. But everything Ops-related should absolutely be transparent to Devs and bring little to 0 noise.
Personally, I prefer to focus on writing the application code and be sure all the client’s features are implemented correctly, rather than dwelling into the labyrinths of unreachable networks and unmountable volumes and what not.
Going back to the tests, the XUnit Fixture is basically the heart of the sample:
public class DbFixture : IDisposable { public DbFixture() { var config = new ConfigurationBuilder() .AddJsonFile("appsettings.json") .Build(); var connString = config.GetConnectionString("db"); var dbName = $"test_db_{Guid.NewGuid()}"; this.DbContextSettings = new MongoDbContextSettings(connString, dbName); this.DbContext = new MongoDbContext(this.DbContextSettings); } public MongoDbContextSettings DbContextSettings { get; } public MongoDbContext DbContext { get; } public void Dispose() { var client = new MongoClient(this.DbContextSettings.ConnectionString); client.DropDatabase(this.DbContextSettings.DatabaseName); } }
Let’s see what’s happening here:
- it loads the connection string from the configuration file
- generates a random DB name
- spins up the MongoDbContext
- ensures that the DB is dropped at the end of the suite
Few words on Point 2 and 4:
- Point 2 is extremely important to ensure that every execution is isolated. We don’t want test data created for a class messing with tests in another file.
- The
Dispose()
method gives guarantee for Point 4. Once a test suite is executed there’s no point keeping the DB around.
That’s all for today. With the CoVid still around, stay home, stay healthy, get some vitamins and code!