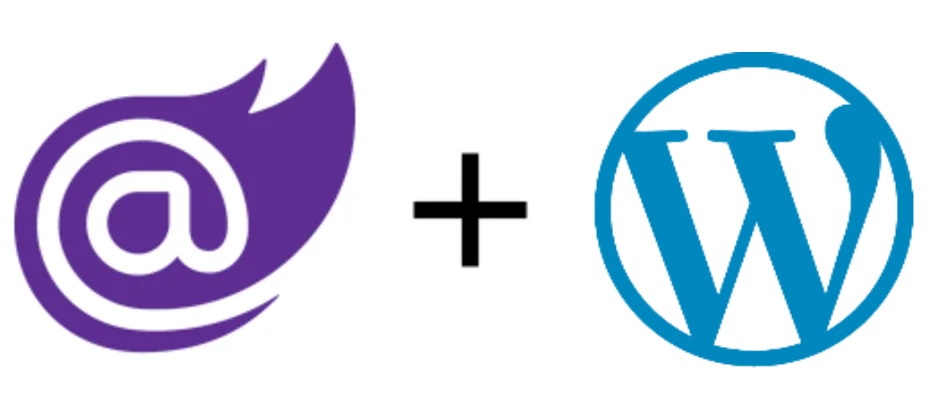
Write a WordPress blog with…Blazor!
Yeah, I’m not getting crazy. It is 100% possible to replace your WordPress theme with a Blazor Webassembly application.
How? Well, it’s not that hard actually. It’s been a while now since WordPress has started exposing a quite nice REST API. The first draft was added with version 4.4, but it’s with version 4.7 that it gained full momentum.
The API itself is definitely easy to use. The base endpoint looks something like “yoursite.com/wp-json/wp/v2“.
From that you can start managing posts, users, comments…well you got the idea.
This opens the door to a wide range of scenarios, for example, we could use WordPress as a headless CMS and write multiple frontends based on our needs. Mobile apps, SPAs, PWAs and so on.
Just to showcase how easy it is, let’s see how we can quickly write a frontend blogging app using Blazor.
Now, CORS should be enabled by default. If it isn’t, a quick search on google should give you the answer. For the sake of the exercise, let’s suppose it is, so we can safely use Blazor in Webassembly mode.
Once we’ve created our project, the next step is to add a reference to the WordPressPCL Nuget library. It’s a handy project, that will spare us the time to write the API client ourselves.
Once it’s installed, we can register the client on the Composition Root in the Program.cs file:
var wpApiEndpoint = builder.Configuration["WP_Endpoint"]; var client = new WordPressClient(wpApiEndpoint); builder.Services.AddSingleton(client);
Now, we’re basically done! Every time we want to, for example, read posts or anything, all we have to do is inject the WordPressClient instance and use it:
var postsQuery = new PostsQueryBuilder() { Page = 1, PerPage = 10, Order = Order.DESC, OrderBy = PostsOrderBy.Date }; var posts = await WPClient.Posts.Query(postsQuery);
I have created a small repo on GitHub as usual with some handy examples. I also added a GitHub Action pipeline that will deploy the code to GitHub Pages, so you can see how it would look like.
For detailed instructions on how to host a Blazor Webassembly application on GitHub Pages, you can take a look at my previous post.
See ya!