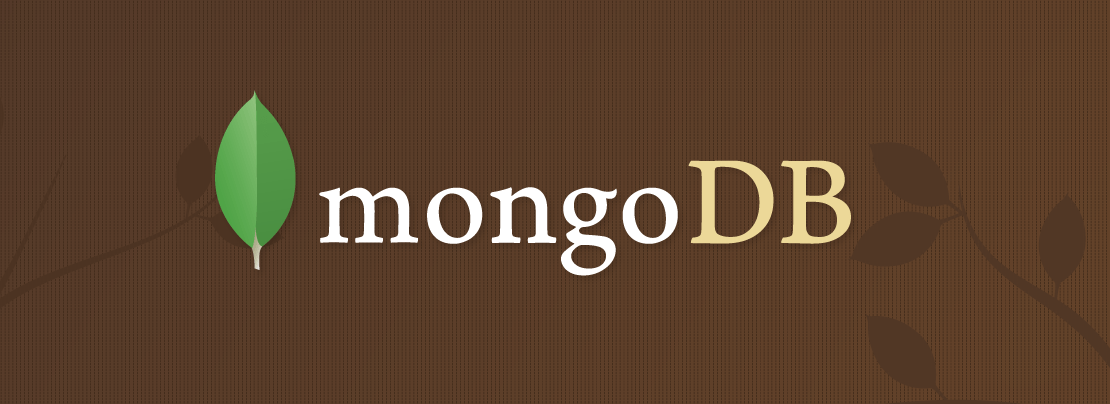
Unit testing MongoDB in C# part 3: the database factories
Welcome to the third article of the series!
Last time I was talking about the database context and at how I injected a Factory to create the repositories. Of course we could have injected every single repository in the cTor, but this way adding a new collection to the database would force too many changes.
Injecting just the factory instead allows us to create internally all the repositories we need, add new ones easily and of course makes our life easier when it comes to testing.
Let’s take a look at our Repository Factory interface:
public interface IRepositoryFactory | |
{ | |
IRepository<TEntity> Create<TEntity>(RepositoryOptions options); | |
} |
as you can see, that’s very standard and easy. The implementation also is pretty straightforward:
public class RepositoryFactory : IRepositoryFactory | |
{ | |
private readonly IMongoDatabaseFactory _dbFactory; | |
public RepositoryFactory(IMongoDatabaseFactory dbFactory) | |
{ | |
if (dbFactory == null) | |
throw new ArgumentNullException("dbFactory"); | |
_dbFactory = dbFactory; | |
} | |
public IRepository<TEntity> Create<TEntity>(RepositoryOptions options) | |
{ | |
if (options == null) throw new ArgumentNullException("options"); | |
var db = _dbFactory.Connect(options.ConnectionString, options.DbName); | |
return new Repository<TEntity>(db.GetCollection<TEntity>(options.CollectionName)); | |
} | |
} |
A couple of notes on this:
- the RepositoryOptions class is just a simple Value Object encapsulating some details like the connection string and the name of the collection
- in the cTor we have a dependency on another Factory used to get a reference to the database. Why we do this? I guess you know the answer 😀
As you can see, this injected Factory also is very easy:
public interface IMongoDatabaseFactory | |
{ | |
IMongoDatabase Connect(string connectionString, string dbName); | |
} |
you can find the implementation here.
Next time: let’s write some tests!