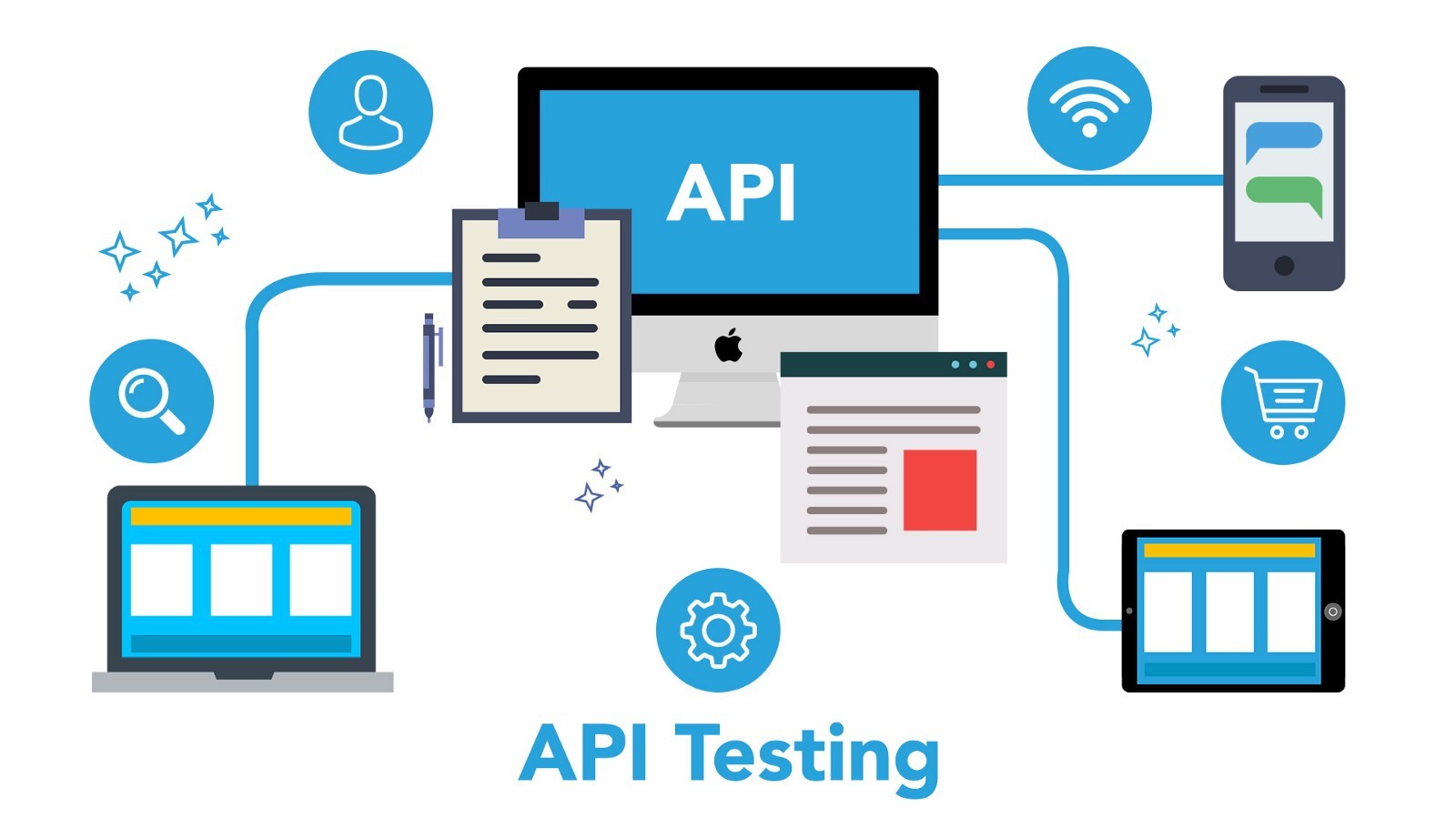
Testing the boundaries of your Web APIs
How do you make sure an entire software you wrote works? And how would you do that if your system doesn’t have a UI? Well, simply by testing the boundaries of course!
From time to time I like to extract pieces of code from what I’m working on and create small repos just to showcase a single functionality or idea.
This time I’m putting some efforts on TDD on APIs and after few refactorings I came up with a nice structure that you can use as a starting skeleton for a simple system. You can find all the sources here on GitHub.
The demo is very simple, just a single controller that stores and provides user details. Nothing fancy. The user model class exposes only three properties: id, full name and email.
Few points worth noting though:
- the class is immutable. I wrote a bit about the concept here.
- I’m adopting the Special Case (or Null Object) pattern a lot these days. Hence the NullUser static property.
Persistence is done in-memory as it’s obviously outside the scope. Moreover, as you can see the Tests project contains only the end-to-end tests, no unit/integration test to cover the persistence layer.
The testing infrastructure is where things gets interesting, even though it’s actually fairly straightforward. An XUnit Fixture is firing up a TestServer and bootstrapping the application using (possibly) the same settings as the real system.
A shared WebHostBuilderFactory class is indeed responsible of building the required IWebHostBuilder instance.
That’s it!
Ok, just to be honest, I got the idea from Mark Seemann : he has a very interesting course on Pluralsight named “Outside-in TDD“. If you have the chance, I strongly suggest you to watch it.
So, now that we have our nice infrastructure ready, all we have to do is write our tests! Being this a Web API, these might be considered either “functional” or “end to end”.
Honestly I think it’s simply a naming thing and doesn’t change the fact that probably these should be the first tests you would write.
Why? Because (and Mark explains it really well in his course) you’re ensuring from the consumer’s perspective that your APIs do what they’re expected to do.
You’ll be “testing the boundaries”.
But most importantly, you’re validating your acceptance criteria and making sure your system works.
Everything else is just an implementation detail.
So what are we testing here? The routes of course! Our API is managing users, and being it RESTful, we’re asserting that all the http verbs are doing what we expect to do.
Most of these tests should derive directly from the acceptance criteria written by your Product Owner. In case you don’t have one but instead rely on some (even vague) specifications, a good starting point is simply testing inputs and outputs.
Happy testing!