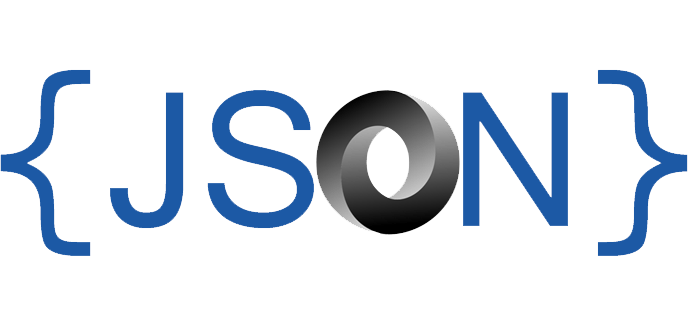
Store and retrieve a class containing interfaces with JSON.NET
Suppose you have code like this:
public interface IMyInterface | |
{ | |
// blah | |
} | |
public class MyClass | |
{ | |
public IEnumerable<IMyInterface> TheItems { get; set; } | |
} |
and you want to serialize in JSON an instance of MyClass. If you are using JSON.NET it is most likely that you will get this error when you try to get the data back:
Newtonsoft.Json.JsonSerializationException: Could not create an instance of type IMyInterface. Type is an interface or abstract class and cannot be instantiated.
Worry you not! The solution is at hand ! Or, at least, you have several options.
- Write a custom serializer and tell the library EXACTLY how you want your data to be written. Depending on your structure and the time at your disposal, this can be a long task.
- Ask the library to encode information about the types directly into the JSON. Yay!
var myClassInstance = new MyClass(); | |
// blah | |
string jsonData = JsonConvert.SerializeObject(myClassInstance, Formatting.Indented, new JsonSerializerSettings | |
{ | |
TypeNameHandling = TypeNameHandling.Objects, | |
TypeNameAssemblyFormat = System.Runtime.Serialization.Formatters.FormatterAssemblyStyle.Simple | |
}); |
the generated json data will contain all the info required to correctly de-serialize the data back. Of course this means that the string will be a little bit longer, so be advised (for more details take a look at the docs here ) ,
To get your instance back you have to tell the library that you have encoded type infos:
var myClassInstaceDeserialized = JsonConvert.DeserializeObject<MyClass>(jsonData, new JsonSerializerSettings | |
{ | |
TypeNameHandling = TypeNameHandling.Objects | |
}); |
cheers 😀