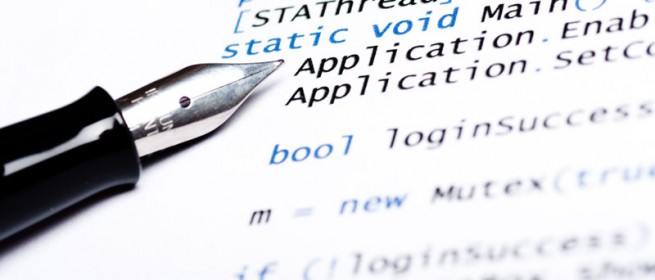
MVC: reading LinkedIn user profile data
Suppose you have to login your users using an external provider. Maybe LinkedIn. Suppose you have to read the user profile data and do some stuff. Maybe you have to register the guy on your website.
Maybe you know a bit of OAuth and you want to give it a try but maybe you don’t want to bother writing a library from scratch.
What do you do? Search on NuGet of course! I have found a nice library that helps with this, named Sparkle.LinkedInNET 🙂
Here’s a quick’N’dirty gist showing the bare minimum necessary to get the oauth token and reading the profile data. Then use it wisely!
As you may imagine, the flow begins with the Profile action ( something like “http://mydomain/linkedin/profile” ). The user will be redirected to LinkedIn and from there back to your website, this time with a token that you can use to interact with the LinkedIn APIs.
Here’s the full code, enjoy 🙂
public class LinkedInController : Controller | |
{ | |
public RedirectResult Profile() | |
{ | |
var redirectUrl = "http://mydomain/linkedin/profilereturn/"; | |
var url = GetAuthorizationUrl(redirectUrl); | |
return Redirect(url.ToString()); | |
} | |
public ActionResult ProfileReturn(string code, string state) | |
{ | |
var redirectUrl = "http://mydomain/linkedin/profilereturn/"; | |
var profile = ReadMyProfile(code, redirectUrl); | |
var jsonProfile = Newtonsoft.Json.JsonConvert.SerializeObject(profile); | |
return Content(jsonProfile); | |
} | |
private static Uri GetAuthorizationUrl(string redirectUrl) | |
{ | |
var api = CreateAPI(); | |
var scope = Sparkle.LinkedInNET.OAuth2.AuthorizationScope.ReadBasicProfile | | |
Sparkle.LinkedInNET.OAuth2.AuthorizationScope.ReadEmailAddress | | |
Sparkle.LinkedInNET.OAuth2.AuthorizationScope.ReadContactInfo; | |
var state = Guid.NewGuid().ToString(); | |
var url = api.OAuth2.GetAuthorizationUrl(scope, state, redirectUrl); | |
return url; | |
} | |
private static Person ReadMyProfile(string code, string redirectUrl) | |
{ | |
var api = CreateAPI(); | |
var userToken = api.OAuth2.GetAccessToken(code, redirectUrl); | |
var user = new Sparkle.LinkedInNET.UserAuthorization(userToken.AccessToken); | |
var fieldSelector = FieldSelector.For<Person>().WithFirstName() | |
.WithLastName() | |
.WithEmailAddress(); | |
var profile = api.Profiles.GetMyProfile(user, null, fieldSelector); | |
return profile; | |
} | |
private static LinkedInApi CreateAPI() | |
{ | |
var config = new LinkedInApiConfiguration(ConfigurationManager.AppSettings["LinkedIn_AppID"], | |
ConfigurationManager.AppSettings["LinkedIn_AppSecret"]); | |
var api = new LinkedInApi(config); | |
return api; | |
} | |
} |