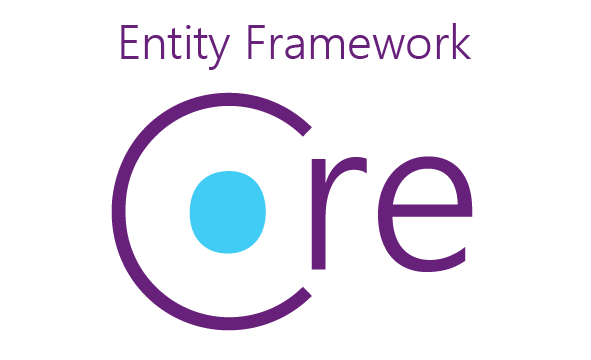
Let's do some DDD with Entity Framework!
Few days ago Microsoft released Entity Framework Core 3, introducing a lot of improvements in both functionalities and performance ( and some breaking change as well).
Now we have full support for very cool stuff like C# 8, Async Enumerables, plus a brand new LINQ provider.
I’m using a lot Entity Framework in my daily job but of course before upgrading a project ‘s dependency to a mayor version there’s always some testing to do.
As I often do, I decided to write a small demo and try out some of these new functionalities. You can find the all sources available on GitHub.
My goal for this project was to model a bunch of Aggregates and Value Objects with proper Persistence Ignorance, eg: I don’t want to pollute my business logic classes with code responsible of storing and retrieving data.
Luckily Entity Framework Core offers the possibility to configure the Entity/Table mapping using a nice Fluent Interface , avoiding attributes on our properties.
We have basically two options. First one is to write all the configuration code in the OnModelCreating() method of our DbContext. Quick and easy, but leads to a lot of very confused code.
A much cleaner option is to use the IEntityTypeConfiguration<> interface. With it we can separate the configuration for each Entity and have a much cleaner structure.
Using this second option, I modeled a very simple eCommerce scenario with Products, Quotes and Orders. These last two Entities also hold one-to-many relationships with Quote Items and Order Lines respectively.
When you run the application the first time, it will generate the db, which looks like this:
<figcaption>database diagram generated with https://sqldbm.com </figcaption></figure>
Then the code will:
- create some products
- add just one product to a quote and save it
- update the quote adding another product
- create an order from that quote
- add another product to the quote
- create another order from the quote
In the next post we’re going to take a look at the code and talk about each Entity configuration. Stay tuned!