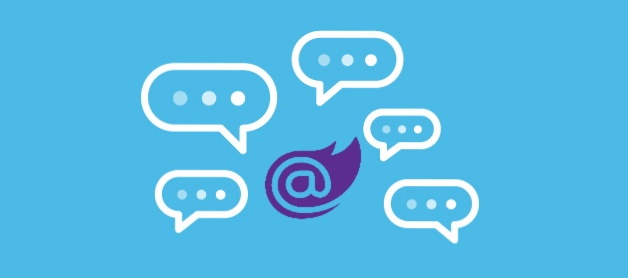
Blazor how-to’s: create a chat application – part 1: introduction
Here we are for another episode of Blazor how-to’s! This time we’ll see how we can easily create a chat application with Blazor and .NET Core.
As usual, all the code is available on GitHub.
Disclaimer: don’t expect anything fancy like Whatsapp or Slack 🙂 Our application will showcase just a bunch of features:
- single chat room
- (extremely) simple authentication
- online status
This is more or less as it will look like:
<figcaption>behold! BlazorChat!</figcaption></figure>
Nothing fancy, I told you 🙂
As you can see, the users can log in using a custom username (no password needed) and talk to each other in a single chat room. A red/green icon is displaying the online status.
Disclaimer 2: the code of the repo is far away from being production ready. We’ll talk more about this later on, but again, don’t expect to plug the cord and have a 100% working messaging system. There’s no DDD, models are quite anemic and everything is in memory.
My main focus was to see how easy it could be to have a simple chat up and running with Blazor only. With that said, it won’t be very complex to refactor the code and make it more robust. But as I wrote before, we’ll talk more about this, eventually.
So, let’s go through the features now!
Single chat room
As already said, the messages are not persisted, meaning that the system will lose everything when rebooting. I have implemented the communication with a bounded Channel wrapped into a simple interface, so it can be swapped with RabbitMQ, for example.
private readonly ChannelWriter<Message> _writer; public async Task PublishAsync(Message message) { await _writer.WriteAsync(message); }
Messages are then pulled from the queue (aka channel ) with a Background Worker. The Consumer will then dispatch an event that will eventually be picked up by the UI:
private readonly ChannelReader<Message> _reader; public async Task BeginConsumeAsync() { await foreach (var message in _reader.ReadAllAsync()) { this.MessageReceived?.Invoke(this, message); } } public event EventHandler<Message> MessageReceived;
That’s all for now! Next time we’ll see how we can “authenticate” our users. Cheers!